Generating a PowerPoint document from scratch is painful and
time consuming, but it would be much easier if you use a template with
pre-designed formats. In this article, I will introduce how to generate PowerPoint
document from existing template in Java using Free Spire.Presentation for Java
library.
Add Dependencies
There are two ways to include Free Spire.Presentation for
Java in your Java project.
For maven projects, add the following dependencies to your
project’s pom.xml file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | <repositories> <repository> <id>com.e-iceblue</id> <name>e-iceblue</name> <url>http: //repo.e-iceblue.com/nexus/content/groups/public/</url> </repository> </repositories> <dependencies> <dependency> <groupId> e-iceblue </groupId> <artifactId>spire.presentation.free</artifactId> <version> 3.9 . 0 </version> </dependency> </dependencies> |
For non-maven projects, download Free Spire.Presentation for
Java pack, extract the
zip file, then add Free Spire.Presentation.jar in the lib folder into your
project as a dependency.
The following is the input PowerPoint template which
contains placeholder text:
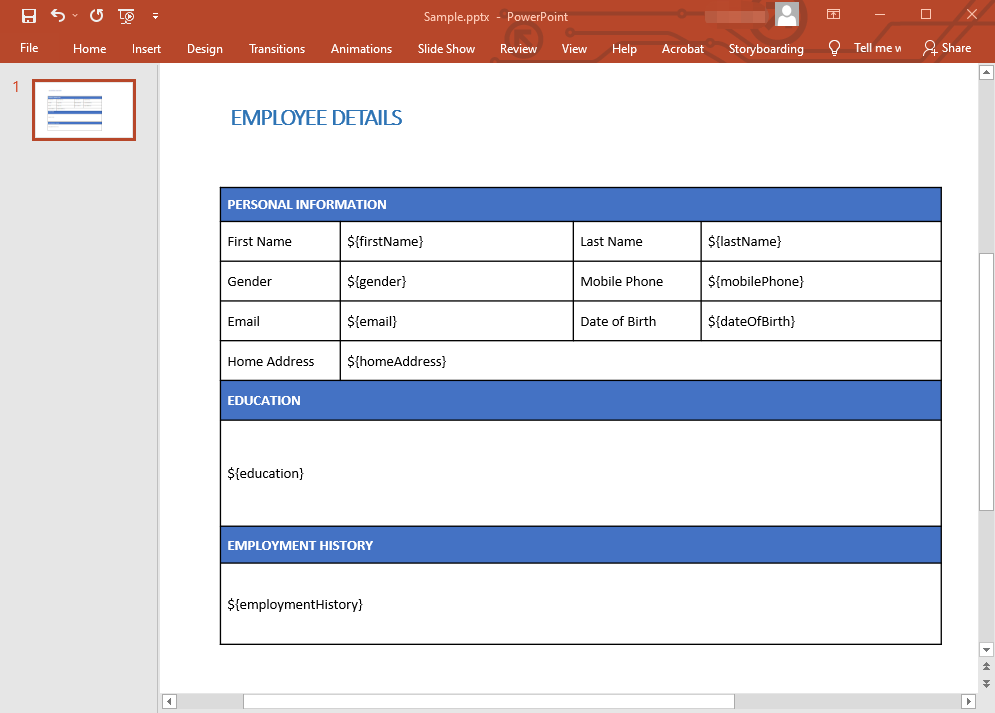
In the following example, I will show you how to generate a
PowerPoint document from the template by replacing the placeholder text.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | import com.spire.presentation.*; import java.util.HashMap; import java.util.Map; public class CreatePptFromTemplate { public static void main(String []args) throws Exception { //create a Presentation instance Presentation presentation = new Presentation(); //load the template PowerPoint document presentation.loadFromFile( "C:\\Users\\Administrator\\Desktop\\Sample.pptx" ); //get the first slide ISlide slide= presentation.getSlides().get( 0 ); //create a map of values for the template Map<String, String> map = new HashMap<>(); map.put( "firstName" , "Alex" ); map.put( "lastName" , "Anderson" ); map.put( "gender" , "Male" ); map.put( "mobilePhone" , "+0044 85430000" ); map.put( "email" , "alex.anderson@myemail.com" ); map.put( "homeAddress" , "123 High Street" ); map.put( "dateOfBirth" , "6th June, 1986" ); map.put( "education" , "University of South Florida, September 2013 - June 2017" ); map.put( "employmentHistory" , "Automation Inc. November 2013 - Present" ); //replace the placeholder text in the template for (Map.Entry<String, String> entry : map.entrySet()) { slide.replaceAllText( "${" + entry.getKey() + "}" , entry.getValue(), true ); } //save the result document presentation.saveToFile( "ReplaceText.pptx" , FileFormat.PPTX_2013); } } |
The following is the output PowerPoint document:
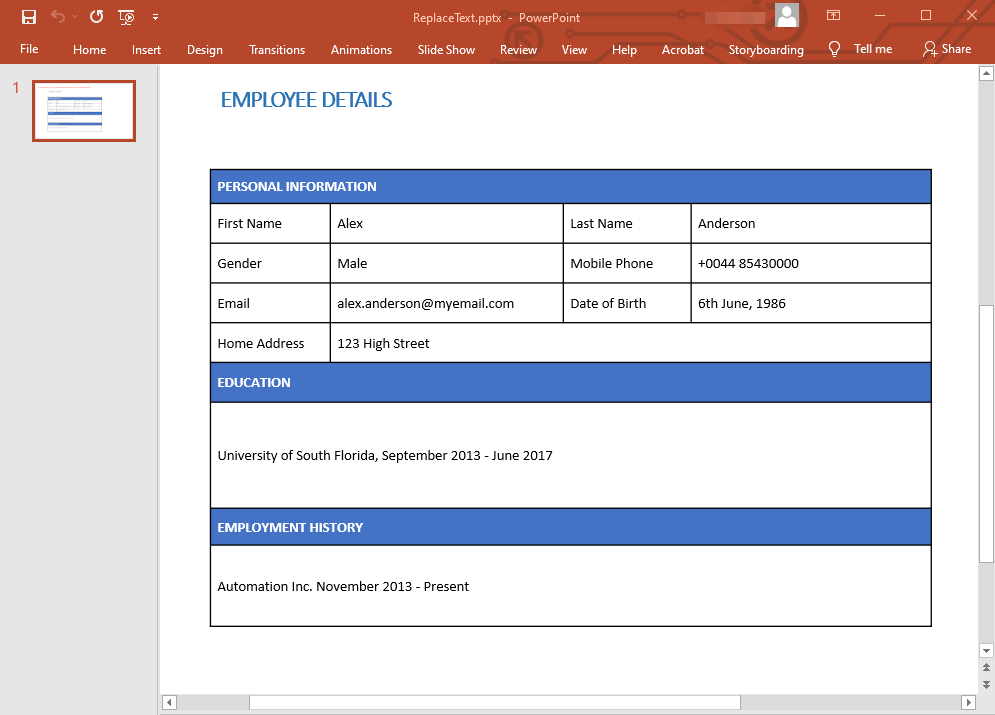
Conclusion
From this example, you can see it’s very easy to deal with
PowerPoint objects with Free Spire.Presentation for Java library. The library provides
rich capabilities to work with PowerPoint documents, you can explore more about
it by visiting the documentation.
Thanks for reading and happy coding!
Comments
Post a Comment