Convert Word document to other file formats is undoubtedly one
of the most common requirements when we working with Word document. In this
article, we’ll learn how to convert Word document to other file formats programmatically
in Java by using Free Spire.Doc for Java library.
Free Spire.Doc for Java library let us convert Word document
to a series of file formats easily and effectively without having Microsoft
Office to be installed on our system. The following conversions are supported
by Free Spire.Doc for Java:
- Microsoft Word file format conversions
- Convert Word to Pdf
- Convert Word to Image
- Convert Word to Html
- Convert Word to Rtf
- Convert Word to Svg
- Convert Word to Txt
- Convert Word to Xps
- Convert Word to Pcl
- Convert Word to Post Script
- Convert Word to Odt
- Convert Word to Epub
- Convert Word to WordML/WordXML
In the following examples, we’ll see how to convert Word
document to Pdf, Image, Html and Svg.
Convert Word to Pdf
The following example demonstrates how to convert a Word
document to Pdf file format.
public class ConvertWordToPDF
{
public static void main(String[] args){
Document doc = new Document("Input.docx");
public static void main(String[] args){
Document doc = new Document("Input.docx");
doc.saveToFile("Output.pdf", FileFormat.PDF);
}
}
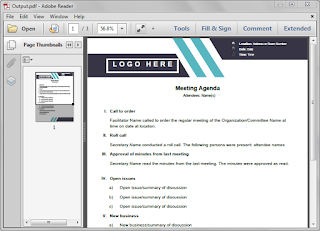
Free Spire.Doc for Java also allows us to convert Word to Pdf
with custom settings, such as whether to embed fonts, whether to disable link,
as shown in below code.
public class ConvertWordToPDF { public static void main(String[] args){ Document doc = new Document("Input.docx"); ToPdfParameterList parameterList = new ToPdfParameterList(); parameterList.isEmbeddedAllFonts(true); parameterList.setDisableLink(true);
doc.saveToFile("Output.pdf", parameterList); } }
Convert Word to Image
Free Spire.Doc for Java supports converting a specific page
of a Word document to an image as well as converting the whole document to
images. The following example shows how to convert the first page of a Word
document to image.
public class ConvertWordToImage { public static void main(String[] args){ Document doc = new Document("Input.docx");
BufferedImage image= doc.saveToImages(0, ImageType.Bitmap);
File file= new File("Output.png"); try { ImageIO.write(image, "PNG", file); } catch (IOException e) { e.printStackTrace(); } } }
Convert Word to Html
The following example shows how to convert a Word document
to Html file format.
public class ConvertWordToHTML { public static void main(String[] args){ Document doc = new Document("Input.docx");
doc.saveToFile("Output.html", FileFormat.Html); } }
Convert Word to Svg
The following example illustrates how to convert a Word
document to Svg file format.
public class ConvertWordToPDF { public static void main(String[] args){ Document doc = new Document("Input.docx"); doc.saveToFile("Output.svg", FileFormat.Svg); } }
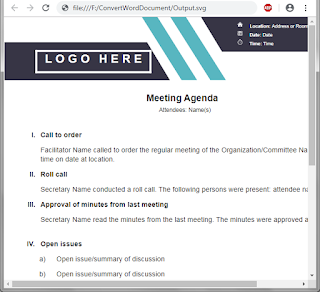
Note: The free version is limited to 3 pages of conversion, if you want to convert more pages, you'll need to upgrade to the professional version of Spire.Doc for Java .
More information
Documentation: https://www.e-iceblue.com/Tutorials/Java/Spire.Doc-for-Java/Program-Guide/Spire.Doc-Program-Guide-Content-for-Java.html
Support forum: https://www.e-iceblue.com/forum
Comments
Post a Comment